发布日期: 2020-06-29 15:29:53
作者: Stephen
评论: 0
微信小程序支付流程:
- 小程序登录,获取openid
- 后端调用微信支付统一下单
- 得到预支付交易会话标识prepay_id
- 组装小程序支付所需的几个参数,并进行签名
- 支付成功,主动查询订单支付状态
- 接收微信支付回调通知
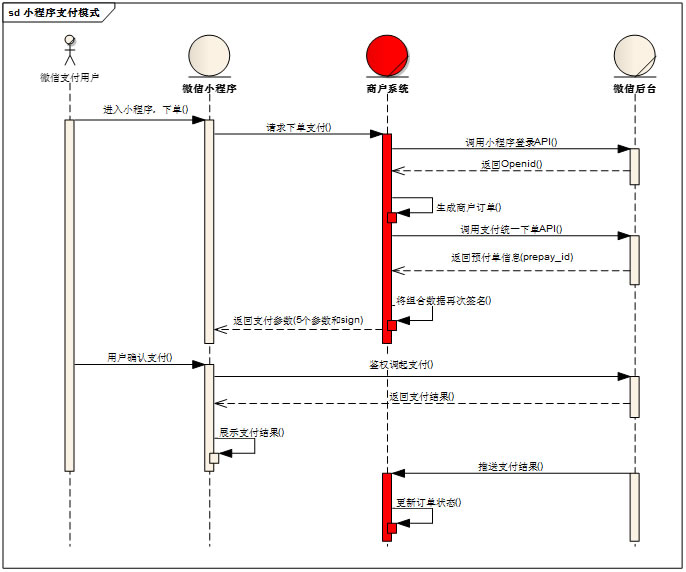
微信小程序支付:
<?php
/**
* 微信小程序支付
* @author Stephen
*/
namespace App\Helper\Pay;
use GuzzleHttp\Client;
class WxApp
{
private $app_id;
private $secret;
private $mch_id;
private $wx_pay_url = 'https://api.mch.weixin.qq.com';
private $pay_uri = '/pay/unifiedorder';
private $order_query_uri = '/pay/orderquery';
public function __construct()
{
$this->app_id = 'app_id';
$this->secret = 'secret';
$this->mch_id = 'mch_id';
}
/**
* 下单
* @param float $totalFee
* @param string $orderNo
* @param string $orderName
* @param string $notifyUrl
* @param string $openId
* @return array
* @throws \GuzzleHttp\Exception\GuzzleException|\Exception
*/
public function pay(float $totalFee, string $orderNo, string $orderName, string $notifyUrl, string $openId) : array
{
$data = [
'appid' => $this->app_id,
'mch_id' => $this->mch_id,
'nonce_str' => $this->generateNonceStr(),
'sign_type' => 'MD5',
'body' => $orderName,
'out_trade_no' => $orderNo,
'fee_type' => 'CNY',
'total_fee' => intval($totalFee * 100), // 单位:分
'spbill_create_ip' => request()->getClientIp(),
'time_start' => date('YmdHis'),
'notify_url' => $notifyUrl,
'trade_type' => 'JSAPI',
'openid' => $openId
];
$data['sign'] = $this->generateSignature($data);
$payXmlStr = $this->arrayToXml($data);
$unifiedOrder = $this->request($this->pay_uri, $payXmlStr);
if (!$unifiedOrder) {
throw new \Exception('支付请求失败');
}
if ($unifiedOrder['return_code'] != 'SUCCESS') {
throw new \Exception($unifiedOrder['return_msg'] ?? '支付请求失败');
}
if ($unifiedOrder['result_code'] != 'SUCCESS') {
throw new \Exception($unifiedOrder['err_code'] ?? '支付请求失败');
}
return $this->getWxPayData($unifiedOrder);
}
/**
* 回调
* @return string
* @throws \Exception
*/
public function notify($notify)
{
if (!$notify) {
throw new \Exception('Parse XML Error');
}
if ($notify['return_code'] != 'SUCCESS') {
throw new \Exception($notify['return_msg']));
}
if ($notify['result_code'] != 'SUCCESS') {
throw new \Exception($notify['err_code']));
}
//签名验证
$signature = $notify['sign'];
$array = (array)$notify;
unset($array['sign']);
if ($signature != $this->generateSignature($array)) {
throw new \Exception('签名验证失败');
}
return '<xml><return_code><![CDATA[SUCCESS]]></return_code><return_msg><![CDATA[OK]]></return_msg></xml>';
}
/**
* 查询订单
* @param string $orderNo
* @param string $wxOrderNo
* @return \SimpleXMLElement
* @throws \GuzzleHttp\Exception\GuzzleException
*/
public function query(string $orderNo, ?string $wxOrderNo='')
{
$data = [
'appid' => $this->app_id,
'mch_id' => $this->mch_id,
'out_trade_no' => $orderNo,
'nonce_str' => $this->generateNonceStr(),
'sign_type' => 'MD5'
];
$wxOrderNo && $data['transaction_id'] = $wxOrderNo;
$data['sign'] = $this->generateSignature($data);
$payXmlStr = $this->arrayToXml($data);
$unifiedOrder = $this->request($this->order_query_uri, $payXmlStr);
if (!$unifiedOrder) {
throw new \Exception('订单查询失败');
}
if ($unifiedOrder['return_code'] != 'SUCCESS') {
throw new \Exception($unifiedOrder['return_msg'] ?? '订单查询失败');
}
if ($unifiedOrder['result_code'] != 'SUCCESS') {
throw new \Exception($unifiedOrder['err_code'] ?? '订单查询失败');
}
return $unifiedOrder;
}
/**
* 生成随机字符串
* @param int|null $length
* @return string
*/
private function generateNonceStr(?int $length = 16)
{
$chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789';
$str = '';
for ($i = 0; $i < $length; $i++) {
$str .= substr($chars, mt_rand(0, strlen($chars) - 1), 1);
}
return $str;
}
/**
* 生成签名
* @param array $params
* @return string
*/
private function generateSignature(array $params)
{
ksort($params, SORT_STRING);
$formatStr = $this->formatQueryStr($params);
return strtoupper(md5($formatStr));
}
private function formatQueryStr(array $params)
{
$formatStr = "";
foreach ($params as $key => $val) {
$formatStr .= "{$key}={$val}&";
}
return $formatStr."key=".$this->secret;
}
private function arrayToXml(array $params)
{
$xml = "<xml>";
foreach ($params as $key => $val) {
if (is_numeric($val)) {
$xml .= "<" . $key . ">" . $val . "</" . $key . ">";
} else
$xml .= "<" . $key . "><![CDATA[" . $val . "]]></" . $key . ">";
}
$xml .= "</xml>";
return $xml;
}
/**
* 微信小程序调用微信支付的参数
* @param $unifiedOrder
* @return array
*/
private function getWxPayData($unifiedOrder) : array
{
$data = [
'appId' => $this->app_id,
'timeStamp' => time(),
'nonceStr' => $this->generateNonceStr(),
'package' => "prepay_id=" . $unifiedOrder['prepay_id'],
'signType' => 'MD5'
];
$data['paySign'] = $this->generateSignature($data);
return $data;
}
/**
* 微信支付请求
* @param string $url
* @param string|null $method
* @param string|null $body
* @return null|\SimpleXMLElement
* @throws \GuzzleHttp\Exception\GuzzleException
*/
private function request(string $url, string $params)
{
$url = $this->wx_pay_url . $url;
$client = new Client(['verify' => false]);
$response = $client->request('POST', $url, [
'body' => $body
]);
$code = $response->getStatusCode();
if ($code === 200) {
$content = $response->getBody()->getContents();
return simplexml_load_string($content, 'SimpleXMLElement', LIBXML_NOCDATA);
}
return null;
}
}
发起支付请求
$totalPrice = 100; //总金额,单位:元
$orderNo = date('Ymd') . rand(10000, 99999); // 订单号
$proName = '产品名称';
$callback = 'https://xx.com/pay/wxapp/callback'; // 支付成功后回调Url
$openId = ''; // OPEN ID
$wxAppPay = new App\Helper\Pay\WxApp();
// 将支付请求参数直接返回给前端,前端再到微信发起支付请求
return $wxAppPay->pay($totalPrice, $orderNo, $proName, $callback, $openId);
支付完成,主动查询订单支付状态
$orderNo = $_POST['order_no'];
$wxAppPay = new App\Helper\Pay\WxApp();
$response = $wxAppPay->query($orderNo);
if ($response['trade_state'] === 'SUCCESS') {
// todo 支付成功,修改订单状态
}
支付回调
$post = file_get_contents('php://input');
$notify = simplexml_load_string($post, 'SimpleXMLElement', LIBXML_NOCDATA);
$totalFee = $notify['total_fee']; // 总金额
$cashFee = $notify['cash_fee']; // 支付金额
$outOrderId = $notify['transaction_id']; // 微信订单号
$orderId = $notify['out_trade_no']; // 订单号
$paymentTime = $notify['time_end']; // 完成支付时间
// 回调验签
$wxAppPay = new App\Helper\Pay\WxApp();
$response = $wxAppPay->notify($notify);
// todo 完成支付,处理订单